PNG Image Format
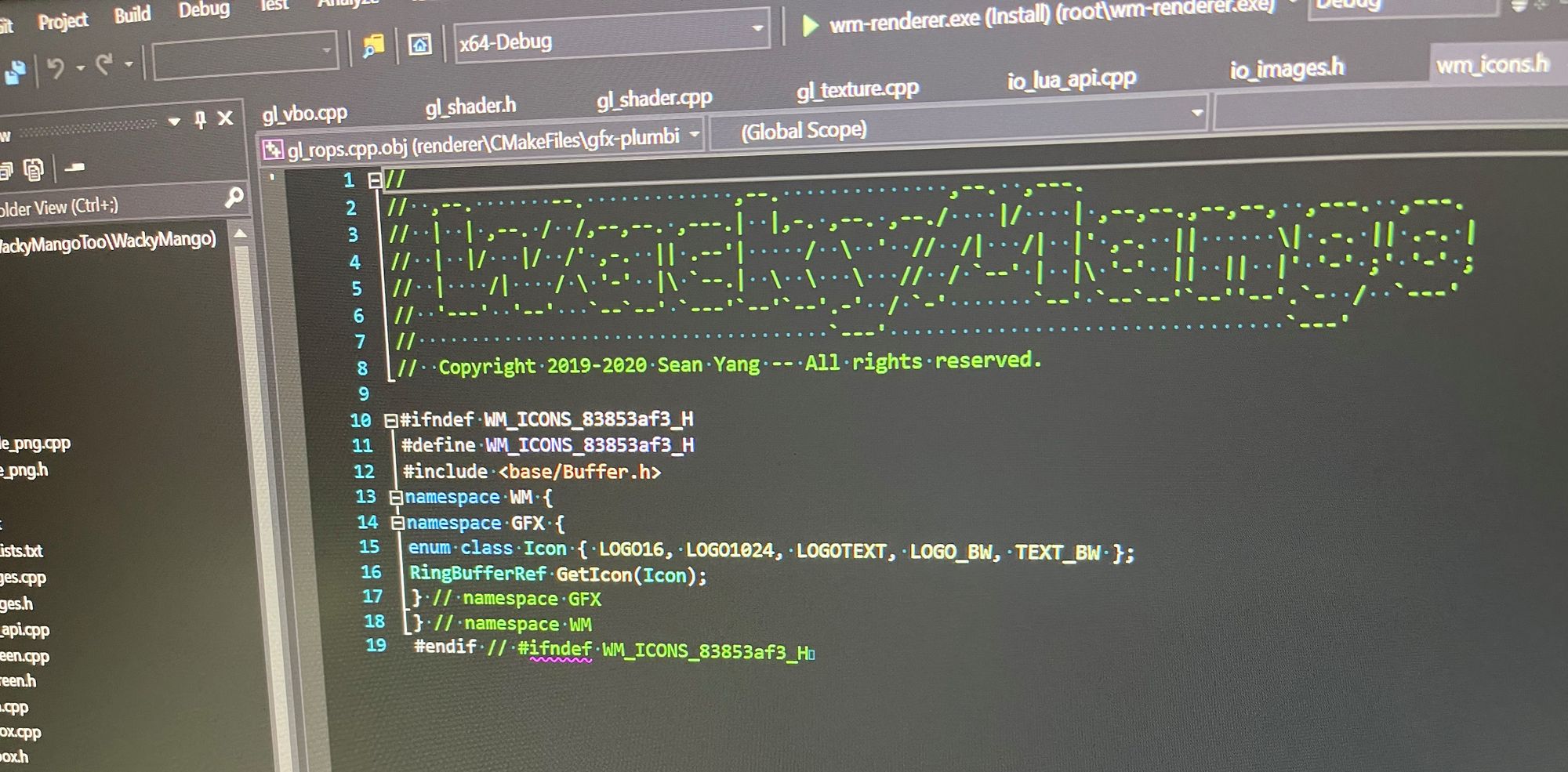
PNG is an image format commonly used where lossless encoding with alpha information is required. It does have some issue with inconsistent gamma correction in some context but is never the less a popular format to support.
License and Source
You can use libpng
to work with this format; source archive is available here. libpng
is Open Source, license is available here. If encoding is not required, a good alternative is libspng
that has a liberal BSD license, available here.
Header and Data Structure
Decoding PNG with libspng
is simple and thread-safe if you don't share the context. All you need for decoding is:
#include <spng.h>
If you want to use libpng
, or use its function to check if you have a PNG file:
#include <png.h>
Format Identification
Using function from libpng
:
if (png_check_sig(p, 8)) {
// First 8-bytes matched PNG signature, you have a PNG image.
}
Decode Code:
Using libspng
:
auto ctx = spng_ctx_new(0 /* flags */);
// buf points to compressed PNG data, etc.
spng_set_png_buffer(ctx, buf, buf_size);
// figure out image dimensions: ihdr.width and ihdr.height.
struct spng_ihdr ihdr;
spng_get_ihdr(ctx, &ihdr);
// out_size tells you size of storage you must allocate for pixel data.
size_t out_size = 0;
spng_decoded_image_size(ctx, SPNG_FMT_RGBA8, &out_size);
// allocate buffer to hold decoded pixels.
auto out = malloc(out_size);
spng_decode_image(ctx, out, out_size, SPNG_FMT_RGBA8, SPNG_DECODE_TRNS);
// after you are done with pixels, liberate memory and context.
free(out);
spng_ctx_free(ctx);
API calls return 0
if successful. Use spng_strerror()
on error code to get human-readable errors.
References
- Wikipedia entry for PNG.
- Documentation for
libpng
. - Documentation for
libspng
.